摘要
web开发一直是行业热门技术,而要做web程序就离不开http服务器,现在主流的http服务器用得最广的如tomcat,apache。还有商用版本的各式各样的http服务器,而在行业类各种微服务,各种web开发技术层出不穷,都是基于这些服务器上的架构上的使用,并没有从本质上提高服务器运行的效率,笔者在研究http服务的过程中,就花了一早上来写了这样一个http服务器展示http服务器工作的流程。
一、什么是http服务器
HTTP服务器一般指网站服务器,是指驻留于因特网上某种类型计算机的程序,可以处理浏览器等Web客户端的请求并返回相应响应,也可以放置网站文件,让全世界浏览;可以放置数据文件,让全世界下载。1目前最主流的三个Web服务器有Tomcat、Apache、 Nginx 。
http服务器用来干什么:
如下图所示,网络通过一个如tomcat一样的http服务器来获取一台计算机上的资源。计算机上的资源可以是计算机能提供的任何服务(包括文件,存储,计算能力等等)。
下图是tomcat中一个http请求的传递过程你,该图在tomcat官网可以找到。
一个请求到响应主要经过了tomcat的Engine->Host->Pipeline->执行servece方法响应http请求。
二、如何自己简单实现一个http服务器
一个简单的实现思路如下,最简单的过程通俗地说就是,请求进来,请求处理器将响应的结果生产出来,再有服务器发出响应。推荐:Java进阶资源
思路上一定是很简单的,实现一个良好可靠的http服务器确实一件很难的事。有很多复制的问题需要解决,如下:
- 什么样的请求能进来或者说是有效的?(第一层请求拦截)
- 请求的解析和响应结果的生成?(需要精通http协议)
- io模型如何选择,怎样充分利用发挥服务器性能?(涉及多线程,复杂的io模型)
- 可插拔要怎么实现?如tomcat可以通过一个servlet让开发人员进行处理。
- …等等,实现一个http服务器绝对是一件很困难的事,所有网络应用层都是基于这个向上设计的。
三、自己实现的http服务器
说干就干,今早花了一个小时写了一个简单的demo来展示,先看下做出来的效果是什么样的。
先在我项目配置的文件夹下放两张图片
通过浏览器加文件名的方式来访问试试结果:
两张图片现在就可以通过网络来浏览了,怎么样,很神奇对吧,接下来就看下代码是怎么实现的?
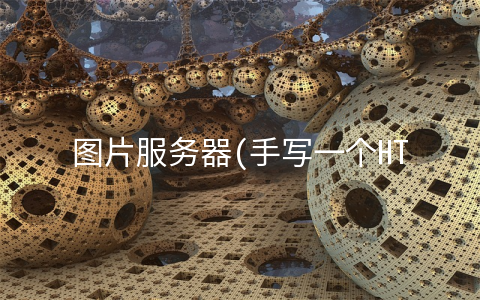
四、Http服务器实现(Java)
HttpServer类:具体的server实现,并且将解析方法也在该类下:
import java.io.*;import java.net.ServerSocket;import java.net.Socket;import java.nio.ByteBuffer;import java.nio.channels.FileChannel;import java.util.Properties;/** * Copyright(c)lbhbinhao@163.com * @author liubinhao * @date 2021/1/1 * ++++ ______ ______ ______ * +++/ /| / /| / /| * +/_____/ | /_____/ | /_____/ | * | | | | | | | | | * | | | | | |________| | | * | | | | | / | | | * | | | | |/___________| | | * | | |___________________ | |____________| | | * | | / / | | | | | | | * | |/ _________________/ / | | / | | / * |_________________________|/b |_____|/ |_____|/ */public class HttpServer { public static final String RESOURCE_PATH = "D:/image"; public static void main(String[] args) throws IOException { ServerSocket server = new ServerSocket(80); while (true){ Socket client = server.accept(); InputStream inputStream = client.getInputStream(); String s = handleNewClientMessage(inputStream); Request request = Request.parse(s); System.out.println(request); //假设请求的都是文件 String uri = request.getUri(); File file = new File(RESOURCE_PATH + uri); FileInputStream fileInputStream = new FileInputStream(file); FileChannel channel = fileInputStream.getChannel(); ByteBuffer buffer = ByteBuffer.allocate((int) file.length()); channel.read(buffer); buffer.rewind(); Response response = new Response(); response.setTopRow("HTTP/1.1 200 OK"); response.getHeaders().put("Content-Type",readProperties(getType(request.getUri()))); response.getHeaders().put("Content-Length",String.valueOf(file.length())); response.getHeaders().put("Server","httpserver-lbh"); response.setBody(buffer.array()); response.setOutputStream(client.getOutputStream()); response.setClient(client); System.out.println(response); System.out.println(client.isClosed()); //响应客户端 response.service(); inputStream.close(); client.close(); } } private static String handleNewClientMessage(InputStream inputStream) throws IOException { byte[] bytes = new byte[1024]; int read = inputStream.read(bytes); StringBuilder buffer = new StringBuilder(); if (read != -1) { for (byte b:bytes) { buffer.append((char)b); } } return buffer.toString(); } /** * 我个人放mimetype文件类型的文件,需要单独修改 */ private static final String MIME_PROPERTIES_LOCATION = "D:\\individual\\springcloudDemo\\src\\main\\java\\contentype.properties"; public static String readProperties(String key) { System.out.println(System.getProperty("user.dir")); Properties prop = new Properties(); try {prop.load(new FileInputStream(MIME_PROPERTIES_LOCATION)); return prop.get(key).toString(); } catch (IOException e) { e.printStackTrace(); } return null; } public static String getType(String uri) { System.out.println("uri:" + uri); String stringDot = "."; String dot = "\\."; String[] fileName; if (uri.contains(stringDot)) { fileName = uri.split(dot); return fileName[fileName.length - 1]; } return null; }}
Request类,请求进来的形式:
import java.util.Arrays;import java.util.HashMap;import java.util.Map;/** * Copyright(c)lbhbinhao@163.com * @author liubinhao * @date 2021/1/1 * ++++ ______ ______ ______ * +++/ /| / /| / /| * +/_____/ | /_____/ | /_____/ | * | | | | | | | | | * | | | | | |________| | | * | | | | | / | | | * | | | | |/___________| | | * | | |___________________ | |____________| | | * | | / / | | | | | | | * | |/ _________________/ / | | / | | / * |_________________________|/b |_____|/ |_____|/ */public class Request { private String method; private String uri; private String version; private byte[] body; private Map<String, String> headers; public String getMethod() { return method; } public String getUri() { return uri; } public String getVersion() { return version; } public byte[] getBody() { return body; } public Map<String, String> getHeaders() { return headers; } public void setMethod(String method) { this.method = method; } public void setUri(String uri) { this.uri = uri; } public void setVersion(String version) { this.version = version; } public void setHeaders(Map<String, String> headers) { this.headers = headers; } public void setBody(byte[] body) { this.body = body; } @Override public String toString() { return "Request{" + "method='" + method + '\'' + ", uri='" + uri + '\'' + ", version='" + version + '\'' + ", body=" + Arrays.toString(body) + ", headers=" + headers + '}'; } private static final String CONTENT_SEPARATE_SIGN = "\r\n\r\n"; private static final String LINE_SEPARATE_SIGN = "\r\n"; private static final String SPACE_SEPARATE_SIGN = "\\s+"; private static final String KV_SEPARATE_SIGN = ":"; //**********************************解析请求************************************* private static void parseTopReq(Request req, String[] topReq){ req.setMethod (topReq[0]); req.setUri (topReq[1]); req.setVersion(topReq[2]); } private static void parseHeaders(Request req, String[] headerStr){ HashMap<String, String> headers = new HashMap<>(12); for (String s : headerStr) { String[] keyValue = s.split(KV_SEPARATE_SIGN); headers.put(keyValue[0], keyValue[1]); } req.setHeaders(headers); } public static Request parse(String reqStr) { Request req = new Request(); String[] httpInfo = reqStr.split(CONTENT_SEPARATE_SIGN); if (httpInfo.length==0){ return null; } if (httpInfo.length > 1){ req.setBody(httpInfo[1].getBytes()); } String[] content = httpInfo[0].split(LINE_SEPARATE_SIGN); // http first row of a http request String[] firstRow = content[0].split(SPACE_SEPARATE_SIGN); // http / get demo_path.type parseTopReq(req, firstRow); parseHeaders(req, Arrays.copyOfRange(content,1,content.length)); return req; }}
Response类,具体响应的结果:
import java.io.IOException;import java.io.OutputStream;import java.net.Socket;import java.util.HashMap;import java.util.Map;/** * Copyright..@lbhbinhao@163.com * Author:liubinhao * Date:2020/12/28 * ++++ ______ ______ ______ * +++/ /| / /| / /| * +/_____/ | /_____/ | /_____/ | * | | | | | | | | | * | | | | | |________| | | * | | | | | / | | | * | | | | |/___________| | | * | | |___________________ | |____________| | | * | | / / | | | | | | | * | |/ _________________/ / | | / | | / * |_________________________|/b |_____|/ |_____|/ */public class Response{ private String topRow; private Map<String, String> headers = new HashMap<>(12); private byte[] body; private int responseCode; private OutputStream outputStream; public String getTopRow() { return topRow; } public void setTopRow(String topRow) { this.topRow = topRow; } public Map<String, String> getHeaders() { return headers; } public void setHeaders(Map<String, String> headers) { this.headers = headers; } public byte[] getBody() { return body; } public void setBody(byte[] body) { this.body = body; } public OutputStream getOutputStream() { return outputStream; } public void setOutputStream(OutputStream outputStream) { this.outputStream = outputStream; } public int getResponseCode() { return responseCode; } public void setResponseCode(int responseCode) { this.responseCode = responseCode; } public static final byte[] LINE_SEPARATE_SIGN_BYTES = "\r\n".getBytes(); public static final byte[] KV_SEPARATE_SIGN_BYTES = ":".getBytes(); private Socket client; public Socket getClient() { return client; } public void setClient(Socket client) { this.client = client; } // 如果头数据里不包含Content-Length: x 类型为Transfer-Encoding:// chunked 说明响应数据的长度不固定,结束符已"\r\n0\r\n"这5个字节为结束符 public void service() throws IOException { outputStream.write(this.getTopRow().getBytes()); outputStream.write(LINE_SEPARATE_SIGN_BYTES); for (Map.Entry<String, String> entry : this.getHeaders().entrySet()){ outputStream.write(entry.getKey().getBytes()); outputStream.write(KV_SEPARATE_SIGN_BYTES); outputStream.write(entry.getValue().getBytes()); outputStream.write(LINE_SEPARATE_SIGN_BYTES); } outputStream.write(LINE_SEPARATE_SIGN_BYTES); outputStream.write(this.getBody()); outputStream.flush(); } @Override public String toString() { return "Response{" + "topRow='" + topRow + '\'' + ", headers=" + headers +// ", body=" + Arrays.toString(body) + ", responseCode=" + responseCode + ", client=" + client + '}'; }}
mimetype文件,用来判断请求的类型,由于文件太大,只复制一部分,格式都一样:
contentype.properties
#mdb=application/x-mdbmfp=application/x-shockwave-flashmht=message/rfc822mhtml=message/rfc822mi=application/x-mimid=audio/midmidi=audio/midmil=application/x-milmml=text/xmlmnd=audio/x-musicnet-downloadmns=audio/x-musicnet-streammocha=application/x-javascriptmovie=video/x-sgi-moviemp1=audio/mp1mp2=audio/mp2mp2v=video/mpegmp3=audio/mp3mp4=video/mp4mpa=video/x-mpgmpd=application/vnd#ms-projectmpe=video/x-mpegmpeg=video/mpgmpg=video/mpgmpga=audio/rn-mpegmpp=application/vnd#ms-projectmps=video/x-mpegmpt=application/vnd
五、总结
实现一个http服务什么是核心呢,就在名字里,就是http协议,http协议本质上就是一个基于文本解析表示特定的语义规则,只要读懂了这些规则就能够理解一个http服务器。
但是要开发一个http服务器这个是远远不够的,还需要高超的编程技艺,个人上述只是一个小demo,看上去好些很简单的样子。在个人github上有一个更加完整的实现,基于nio和多线程,效率更高,实现的更加完善,并且支持断点续传的方式
github地址:
源:binhao.blog.csdn.net/article/details/112059406
评论前必须登录!
注册